GraphQL API - Blue Prism Integration
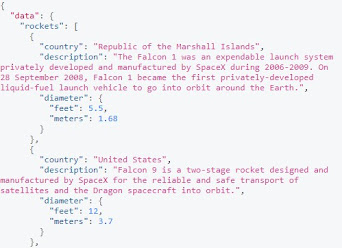
- AddressURL: https://api.spacex.land/graphql/
- JSON: payload
- Result
Started this blog to share my experience as a developer in tools and technologies like RPA, Excel, Outlook, Blue Prism, Rest API, SQL, MySQL, Python (Flask, Django), JavaScript, Machine Learning, Web-Development.
Url: https://api.spacex.land/graphql/
Method: POST
Headers: {'Content-Type': 'application/json'}
Data: provide your select list in JSON format like this:
{
query:`{
launchesPast {
mission_name
launch_site {
site_name_long
}
}
}`
}
You can quickly check its working by creating the below HTML file:
<html>
<head>
</head>
<body>
<button onclick="callAPI()"> Execute </button>
<p id="response-box"></p>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script>
function callAPI(){
var url="https://api.spacex.land/graphql/";
var method="POST"
var body=JSON.stringify({ query:`{
launchesPast {
mission_name
launch_site {
site_name_long
}
}
}`});
var headers={
'Content-Type': 'application/json'
}
console.log("execution started");
$.ajax({
'method': method,
'url': url,
'headers':headers,
'data':body,
'success': function(data){
document.getElementById("response-box").innerHTML = JSON.stringify(data,undefined,2);
}
});
}
</script>
</body>
</html>
The output of the HTML page looks like this:
On clicking the execute button, a javascript function associated with the button triggers the API call. The response from the API is in JSON format as follows:
CRUD stands for Create, Read, Update, Delete which are most basic operations performed on any database. In REST API, we interact with database server using HTTP methods like GET, POST, PUT, DELETE etc,. to perform these operations. While dealing with any API, authentication plays a key role in restricting the access to authorized users. In the following example, I am using a token based authentication(JWT token to be more precise).
I am going to use two database tables to demonstrate all the 4 CRUD Operations of REST API using the concept of Token-based authentication.
For this I am using a free rest API:
Base url: https://restapiplayground.herokuapp.com
Let's understand the table structure before writing or reading data using API.
Database Schema:
There are two tables -
1.User
2.Todo
Schema of the User table looks like this:
And schema of the Todo table looks like this:
Most of the time, we have the need to test the functionality of an API without a third-party tool, as installing an API testing software is not always allowed in an organization.
As we all know, web applications that interact with APIs generally use technologies like javascript, jquery, Ajax to do all the CRUD operations(Create, Read, Update, Delete) on data within the website.
Since web browsers are able to execute the javascript, no other software is required to be installed on the machine in order to query an API.
Let's get started with a small piece of code that can get the API data at the click of a button.
To demonstrate this, I am using the below public rest API:
http://jsonplaceholder.typicode.com/users/1
you can simply browse the above url to see the JSON data which we are going to fetch using our script.
Implementation: Create an HTML file TestApi.html as shown below and run it with any web browser.
1. Get Request (Without Authentication):
TestApi.html
<html>
<head>
<title>Test API with JavaScript</title>
<script>
function callAPI(){
var url="http://jsonplaceholder.typicode.com/users/1";
var method="GET";
var xhreq=new XMLHttpRequest();
xhreq.onload=function(){
document.getElementById("userdata").innerHTML = this.responseText;
}
xhreq.open(method,url);
xhreq.send();
}
</script>
</head>
<body>
<br>
<center>
Click the button get json data<br><br>
<button onclick="callAPI()" class="btn" style="background-color:green; color:white;"> Get Data </button>
</center>
<p id="userdata"></p>
</body>
</html>
The output of the above code looks like this:
After clicking the Get Data Button, json data is loaded on the web page as shown below: The output json data we are expecting is displayed on the screen.That's it.Thank you!
We can use a simple formula to do this real quick.
Let’s say we have two columns data like this.
Now, we can write the formula in the C2 Cell as =A1=B1
Then Press enter and then drag the formula down until where you want the result. The columns is filled with TRUE if the value in the first column matches with value in the second column other wise FALSE.
This is the output of the formula.
Comparing two excel sheets for differences:
We can compare two excel Sheets for differences without any third party tool, just with the help of well known formulas.
This is achieved with the help of conditional formatting.
Steps:
1.Open the the workbook
2. Select the area of the worksheets in both of the excel files
Let's say Sheet1 looks like this.
And Sheet2 looks like this
There are some differences in both the sheets and we want to highlight those differences.
For this we are using conditional formatting formula for the selected ranges.
Click on conditional formatting on Home Tab. Select New Rule
Now Select "Use a formula to determine which cells to format" and Write the formula in the Edit box as =A1<>Sheet1!A1
Then click on Format button to select formatting options for highlighting. Here I want to highlight the differences in RED Colour. Select the colour you want and click OK.
Now the selected colour appears under formula like this.
4. Then click OK, the differences are highlighted with the format specified in the previous step.
Now you are able to see the differences highlighted in the red color.
GraphQL API - Blue Prism Integration This post discusses how to integrate GraphQL API with Blue Prism using a sample graphQL API and Utilit...